This page includes sections Proposed Platform Design, Technology Stack, Backend Express Endpoints, MongoDB Database, External Chat System – Chat Engine, Recommendation System Scoring, Management of User Profile Uploads, and Assumptions. Proposed Platform Design first introduces the design of the overall platform and specific features. Technology Stack describes the technology used in website development and the architecture of Tutor Today. The remaining subsections document specific programming designs chosen during development with Assumptions listing the assumptions made during design.
Proposed Platform Design
To directly address the issues associated with existing platforms, the following designs are presented.
Tutor Today is designed as a web-based format, accessible through web browsers. Users are categorized into two main groups: – “tutors” and “students”. Creating an account is mandatory for users to fully utilize the platform, with browsing being the only available action for non-registered users.
Separate web pages are developed for tutors and students, providing distinct interfaces for each tailored to their specific needs. The tutor interface includes functionalities such as accepting direct and general requests, profile management, location-and-subject preference settings, material listing, and communication channels to connect with students. The student interface provides features such as browsing tutor profiles, requesting direct and all tutors, matching with accepted general requests from tutors, accessing study materials, and engaging in real-time communication with tutors. Designing distinct web pages for tutors and students ensures an intuitive user experience, enabling both parties to utilize all available features effectively.
Students are recommended tutor profiles through an algorithm on their “Tutors” pages and can request specific tutors based on their preferences. They also have the option to make general requests for tutors in particular subjects or categories. A personalized recommendation algorithm takes into account factors such as student needs, location, and ratings from previous students to suggest tutor profiles that best match the individual requirements.
Tutors have the flexibility to accept specific or general tutoring requests. Once a tutor accepts a direct request, they are paired with the student (see Figure 1). This approach allows students to select tutors based on their preferences and requirements, while also allowing tutors to accept requests that align with their expertise and availability, optimizing the matching process. On the other hand, when a tutor accepts a direct request, the student has to also match with the tutor before finalizing the match.
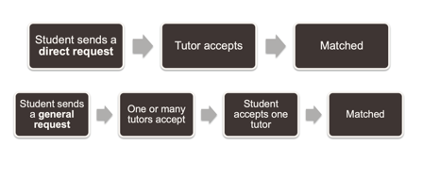
Figure 1: The two processes of requests, with the direct request process on top and the general request process at the bottom.
Tutors on Tutor Today can build detailed profiles showcasing their qualifications, teaching history, and sample exercises. They can upload materials in any file format, providing a preview of the learning materials students can expect in tutorial lessons. This enables students to make informed decisions when selecting a tutor.
Tutor Today implements a rating system where each tutor receives a rating out of 5 stars, solely influenced by reviews from matched students. Students can leave comments on tutor profiles, providing valuable insight to future potential students. Tutors are recommended to students based on their rating. The rating system and profile comments both contribute to transparent feedback, enhancing the credibility of the platform and facilitating informed choices for both tutors and students.
Tutor Today integrates a chat system for instant communication between students and tutors within the platform without the need for a middleman. This ensures security and privacy, as all communication stays within Tutor Today. The chat system facilitates seamless and efficient communication, encouraging users to discuss tutoring needs and share materials, thus improving the tutoring experience.
In the Tutor Today chat system, shared files are automatically stored in a dedicated virtual folder for that chat room in the external chat system, Chat Engine. This centralized file system is accessible to both students and tutors, who can organize the files shared by creating sub-folders and relocating files within the file system. This streamlines the process of accessing files later on and promotes focus during work and separation from work after leaving the website.
Tutor Today sorts requests and tutor profiles from newest to oldest so that students and tutors are always recommended with the most relevant suggestions. Moreover, the database storing MongoDB supports manual deletion, through which administrators of Tutor Today can input queries to delete outdated requests. This approach ensures that the website remains organized and clutter-free, providing an improved experience for tutors by enabling them to focus on active and relevant cases while avoiding outdated ones.
These design choices aim to optimize matching between tutors and students, promote efficient communication, and enhance the user experience.
Technology Stack
The front end of Tutor Today is developed using React, a JavaScript library renowned for efficiently creating dynamic interactive web-based interfaces. This choice is made to utilize the component-based architecture and the virtual DOM (Document Object Model) implementation of React, which allow better modularity during development and improved efficiency for clients respectively. By employing React, the development process also utilizes website development languages including JavaScript, HTML, and CSS, which ensures familiarity and the availability of online resources.
The back end of Tutor Today is developed using Express, a web application framework designed for Node.js. By adopting Express, the development process benefits from the robust and efficient framework simplifying the creation of server-side applications. Node.js serves as the runtime environment for executing the backend logic, providing a scalable and event-driven platform. The choice of Express and Node.js is motivated by their extensive ecosystem, containing an array of libraries, modules, and tools to facilitate development. Additionally, the use of Node.js ensures compatibility with JavaScript, resulting in a seamless integration between the frontend and backend components of Tutor Today.
MongoDB Atlas is selected as the database for storing application data in Tutor Today. MongoDB Atlas offers a cloud, scalable and flexible solution which aligns with the requirements of the application. The document-based model of MongoDB allows the storage of structured and unstructured data in a “NoSQL” format, providing versatility for different program logic. Furthermore, the extensive query capabilities and rich indexing options of MongoDB contribute to efficient data retrieval and management, improving the efficiency of the database management system.
REST (Representational State Transfer) API (Application Programming Interface) defines the endpoints and methods for clients at the front end to interact with the server at the back end , allowing data to be passed back and forth (see Figure 2). The API handles requests from the front end, retrieves and modifies data in the MongoDB database, and responds with the appropriate data or status codes. This architecture ensures a decoupled and efficient communication flow between the client-side and server-side components of the application.
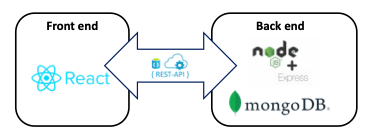
Figure 2: Architecture of Tutor Today. The left side of the figure is the front end of Tutor Today, which is developed with React. The right side of the figure is the back end of Tutor Today, which is developed with Express coupled with Node.js (as the runtime environment). MongoDB in the back end is responsible for storing the application data as the database of the system. Between the front end and back end, REST API allows data transportation between the two.
Backend Express Endpoints
The current back end of Tutor Today connects to MongoDB Atlas and supports API calls for data entry and updates. It utilizes Express to provide different endpoints for API calls, for example, creating or fetching a user in the database and fetching a chat engine user object from the external chat engine. All logic related to the database implemented in the front end is supported, with error handlers catching errors by incorrect API calls. The main endpoints are as follows:
POST method for creating or getting users from Chat Engine:
app.post(“/chatUser/:id”, async (req, res) => {…};
This endpoint takes in the user ID and combines it with the first name and last name of the user, creating the Chat Engine ID. It then calls the Chat Engine to retrieve or create a Chat Engine user. It finally forwards the Chat Engine user as the response.
POST method for creating a new tutor in the database:
app.post(“/tutor”, async (req, res) => { … });
This endpoint takes in the user ID and other user information in the request body, then check if the email and phone number are duplicated. If not, a new tutor record is created in the database. It is used during tutor registration.
POST method for creating a new student in the database:
app.post(“/student”, async (req, res) => {…});
Same as the previous endpoint, this endpoint checks and creates a student record in the database. It is used during student registration.
POST and GET methods for retrieving a registered tutor in the database:
app.post(“/tutor/:email”, async (req, res) => {…});
app.get(“/tutor/:id”, async (req, res) => {
The first and second endpoints take in an email address and tutor ID respectively, and check if a tutor in the database has that email address or ID. If so, it fetches the target tutor record from the database and forwards it as the response. They are used throughout the program to fetch tutor information.
POST and GET methods for retrieving a registered student in the database:
app.post(“/student/:email”, async (req, res) => {…});
app.get(“/student/:id”, async (req, res) => {…});
Same as the previous endpoint, these two endpoints check the database, then retrieve the target student record from the database, and finally forward the record as the response. They are used throughout the program to fetch student information.
GET method for retrieving all requests in the database:
app.get(“/requests/”, async (req, res) => {…});
This endpoint sends all requests in the database as the response. They are used to fetch and display requests for the “Home” and “Requests” pages on both the tutor and student sides.
GET method for retrieving all tutors in the database:
app.get(“/tutors/”, async (req, res) => {…});
This endpoint sends all tutors in the database as the response. It is used to fetch and display tutors in the student “Tutors” page.
POST method for updating a tutor profile:
app.post(“/tutor/updateProfile/:id”, async (req, res) => {…});
This endpoint reads the request body data and updates the target tutor profile accordingly. It is used on the tutor “Profile” page.
POST method for updating a student profile:
app.post(“/student/updateProfile/:id”, async (req, res) => {…});
This endpoint reads the request body data and updates the target tutor profile accordingly. It is used on the student “Profile” page.
POST method for creating a new request:
app.post(“/request”, async (req, res) => {…});
This endpoint reads the body data and creates a direct or general request based on request body data. It also creates a chat room for the tutor and student accordingly. It is used to create requests in the student “Requests” and “Tutors” pages.
POST method for updating a request:
app.post(“/request/:id”, async (req, res) => {…});
This endpoint updates a general or direct request with the target ID based on the request body data. It is used on the tutor “Home” and “Requests” pages when a tutor accepts requests.
POST method for uploading a tutor profile picture to the database:
app.post(“/tutorprofilepic/:id”, async (req, res) => {…});
This endpoint first updates the tutor record to set “profile_pic” to true, then it creates a directory in the back end for this user with his ID as the directory name if there is none. Lastly, it utilizes the library “multer” to save the profile picture in the request body. It is used in the tutor “Profile” page.
POST method for uploading a student profile picture to the database:
app.post(“/studentprofilepic/:id”, async (req, res) => {…});
Same as the previous endpoint, this endpoint saves the profile picture in the database. It is used in the student “Profile” page.
POST method for uploading notes from tutors to the database:
app.post(“/notes/:id”, async (req, res) => {…});
This endpoint creates a directory with the user ID as the name containing another directory called “notes”. It then uploads the note file in the request body to the “notes” directory with the library “multer”. It is in the tutor “Profile” page for uploading notes.
POST method for fetching a resource (profile picture or notes):
app.post(“/resource”, (req, res) => {…});
This endpoint fetches the resource with a path specified in the request body, then sends the resource as a response. It is used throughout Tutor Today to fetch user profile pictures and tutor-uploaded materials.
POST method for uploading a tutor review:
app.post(“/tutorreview/:id”, async (req, res) => {…});
This endpoint uploads a tutor review for the target tutor with the target ID. The review data is read from the request body. It is used on the student “Requests” page to rate tutors.
POST method for updating an accepted general request:
app.post(“/acceptedgeneralrequest/:id”, async (req, res) => {…});
This endpoint updates an accepted request with the target ID based on the request body. It creates a chat room for the tutor and student. It is used in the student “Home” page to allow students to match with a tutor from an accepted general request.
This section explains how the endpoints above support the features in the front end, and form a connection to the MongoDB database and chat system, Chat Engine.
MongoDB Database
This section first covers the rationale of the proposed design of the MongoDB Atlas database, then reveals the implemented database connected to the Express backend system in Tutor Today and the reasons for deviating from the original design.
Proposed Database Design
The design of the database management system supports the storage of application data from clients (see Figure 3). Users are categorized as students or tutors, who are mutually exclusive. They all possess attributes necessary for building profiles and running the tutor profile recommendation algorithm.
Students can make general requests to all tutors, or specific requests to specified tutors as stored in the optional attribute “tutor_id”. After tutors have accepted requests, the attribute status changes to “matched”. All messages hereby after are stored with the mandatory relationship linking it to the matched request. Messages are categorized into text messages and file messages, which are mutually exclusive. Whenever the chat system of a matched request is accessed, the “Text_Message” entity linked to that matched request is accessed and loaded to the front end. Similarly, whenever the file system of a matched request is accessed, the “File_Message” entity linked to that matched request is accessed and built as a virtual file system. To store the ratings of tutors and students in the rating system, reviews are stored in the weak entity linked to that specific tutor or student. The recommendation algorithm processes the data stored, for example, lowering the recommendation score for a tutor review where the derived attribute “recommend” is false. This design enables organized storage of data and allows efficient retrieval of information.
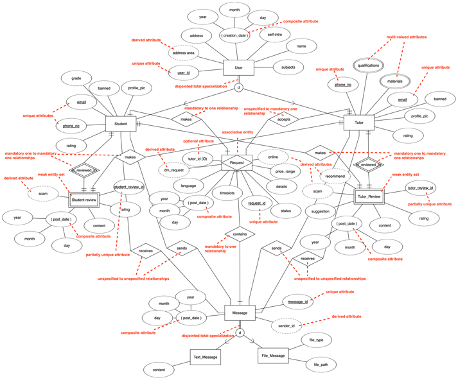
Figure 3: Entity Relationship Diagram of Tutor Today Database Management System [13]. The special types of entities, attributes, and relationships are explained in red. Following the industry convention, entities are in rectangles; attributes are in ovals; and relationships are in rhombuses.
The database management system is fully implemented on MongoDB Atlas (cloud version of MongoDB). Based on the design of the database, there are 5 collections (tables in the database) in total for Tutor Today, including “Requests”, “StudentReviews”, “Students”, “TutorReviews” and “Tutors”. As MongoDB treats data in a NoSQL manner, multi-valued attributes in entities, such as qualifications in the “Tutor” entity, are stored as arrays. Files and pictures in “User” entities are stored as file paths on MongoDB Atlas, as they are planned to be stored as files in the back end.
Implemented Database Design
During the actual implementation, four documents (entities) are deemed excessive and considered retired during development, including “Text_Message”, “File_Message”, “Student_Review” and “Tutor_Review” (see Figure 4).
“Text_Message” and “File_Message” are not needed in Tutor Today, since all chat messages are saved and go through the external chat system, Chat Engine. However, if a more comprehensive file system with more modularity is to be implemented in the future, these two entities are paramount in storing the data internally on MongoDB for more operations.
Apart from the two entities for messages, “Student_Review” and “Tutor_Review” are not used as reviews are currently directly stored at the user entities. MongoDB allows arrays as a filed type, which is used to store all review data. Moreover, only tutor review is chosen to be implemented at this stage, as it is more important for students to gauge tutors than for tutors to gauge students.
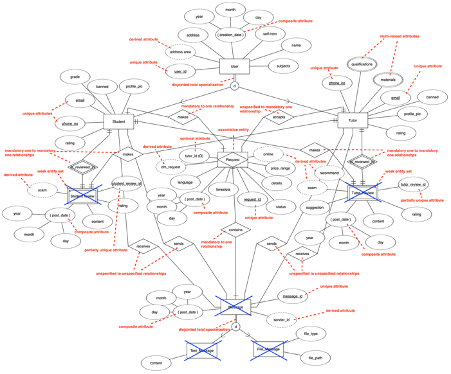
Figure 4: An Entity Relationship Diagram with 5 entities crossed out, representing their retired state.
External Chat System – Chat Engine
The chat system utilizes an external chat engine, called Chat Engine [14]. When a user registers and logs in from the front end of Tutor Today, an API call is sent to the back end. Then, the Express endpoint handles that request by calling the API of Chat Engine, which returns a chat engine user object to the back end. Finally, the back end forwards the chat engine user object to the front end, after which the front end is connected directly to Chat Engine, enabling all chat system functionalities. The chat system supports a variety of functions (see Figure 5), including user navigation into different chat rooms, as well as text, picture, and file exchanges. When a message is sent, for the sender, the chat room displays the timestamp and the users who have read it; for the receivers, a dot is added next to the name of that chat room to indicate there is a new message. The chat system functionalities are further expanded in 4.1. Webpages.
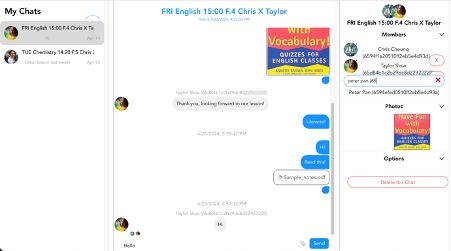
Figure 5: Chat System of Tutor Today, supporting text and file exchanges.
Recommendation System Scoring
After requests and tutors are automatically sorted from latest to oldest when retrieved from the back end, they are scored by the recommendation system.
On the tutor side, each request on the “Home” and “Requests” pages undergoes a scoring process as explained in the pseudo-code in Figure 6. The weighting priorities the subject and the location of the address, and lastly adjusts according to the tuition fee. A score of 10000 is given for a matched interested subject; a score of 2000 for an online class, 1000 for a matched address, 500 for a matched general area (New Territories, Kowloon and Hong Kong Island); a score of (the average of the minimum fee and maximum fee) * duration of the lesson in hours * lessons per week * 1.5. The 1.5 is chosen as the current multiplier which is subject to changes after analyzing more data.
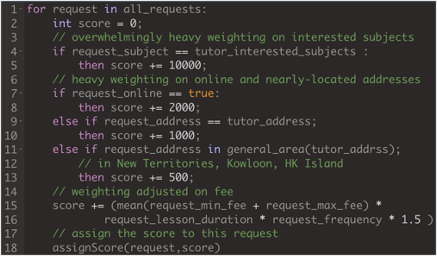
Figure 6: Pseudocode for scoring requests on the tutor side
It should be noted that the tuition fee is not of the lowest priority, as the multiplier allows the tuition fee to make a difference in the sorting process, as seen in the following sample:
The highest weighing on the address score is 2000 points; while a normal tutorial request may have a minimum fee of 300, a maximum fee of 500, 2 hours per lesson, and 2 lessons per week, which is equivalent to (300 + 500) / 2 * 2 * 2 * 1.5 = 2400 points. Hence, even for requests where the location is not ideal, by offering a higher tuition fee, they can be ranked higher with more exposure to tutors.
On the student side, a similar scoring system is implemented for ranking tutor profiles as explained in the pseudo-code in Figure 7. For each matched interested subject (so there can be multiple), a score of 10000 is awarded to that tutor. Then, the same scoring for a matched address applies. This allows the most suited tutor to be ranked at the very top, for example a tutor with the same multiple interested subject as a student.
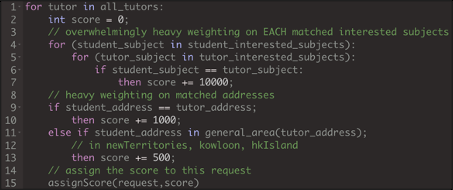
Figure 7: Pseudocode for scoring tutors on the student side.
The two scoring algorithms are a practical alternative to big data analysis, as from experience, there are only a handful of suitable requests for tutors every day and the metrics used in the scoring system stem from real concerns from tutors.
Management of User Profile Uploads
Profile pictures and materials uploaded by tutors in the “Profile” pages are stored directly in the backend inside the directory “Uploads”, within which the file structure in Figure 8 is used to manage user uploads. For each user, a directory with his user ID is created. Inside, his profile picture is saved with the name “profilepicture.jpeg” alongside a directory called “notes”. Inside “notes”, all the materials uploaded by that tutor (students cannot upload any files in their “Profile” pages) are stored with the original file name. There is no limitation on the number nor file type of the files.
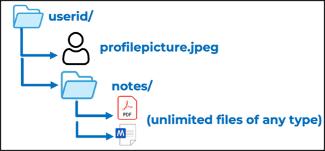
Figure 8: Illustration of the file structure used to store user profile uploads in the directory “uploads” at the back end.
Assumptions
New users are required to provide a phone number during the sign-up process of Tutor Today. It is assumed that acquiring a phone number in Hong Kong involves registering the real name of the person. Therefore, by linking accounts to verified phone numbers, the platform can have a higher level of confidence in the authenticity of user identities, ensuring a trusted and secure environment.
It is also assumed that students on Tutor Today possess the ability to pay tutors through traditional cash-based methods or digital payment platforms without the aid of the platform. Tutor Today does not control nor support the financial transactions between students and tutors but provides freedom for tutors and students to select their preferred payment methods.
Tutor Today operates under the assumption that all users are with no malicious intent, as all files uploaded to the back end are not scanned for viruses. Tutors are free to upload any type of teaching materials including malware. They may corrupt and break the backend of Tutor Today, or be downloaded by unsuspected students.